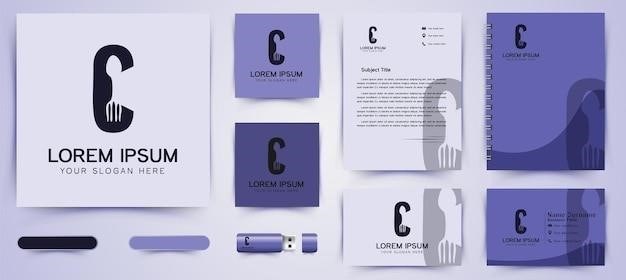
Modern CMake for C++⁚ A Comprehensive Guide
Dive into the world of modern CMake for C++ with this comprehensive guide․ Learn how to build‚ test‚ and package your projects efficiently‚ leveraging the latest features of CMake․ Discover best practices for project structure and code organization‚ explore advanced techniques like external dependencies and conditional compilation‚ and gain insights from real-world applications․ This guide will equip you with the knowledge and skills to master CMake for professional-level C++ development;
Introduction
In the realm of modern C++ development‚ CMake has emerged as the de facto standard for build system automation․ Its ability to manage complex projects‚ streamline compilation processes‚ and enable cross-platform compatibility makes it an indispensable tool for developers of all levels․ This comprehensive guide will equip you with the knowledge and skills to leverage the power of modern CMake for your C++ projects‚ empowering you to build‚ test‚ and package your software with unparalleled efficiency and flexibility․ We will delve into the intricacies of CMake‚ exploring its core features‚ advanced techniques‚ and best practices to ensure that you can confidently create robust and maintainable C++ projects․
Key Features of Modern CMake
Modern CMake boasts a rich set of features designed to simplify and enhance the development workflow for C++ projects․ Its ability to handle complex dependencies‚ generate build systems for various platforms‚ and provide robust testing capabilities makes it a powerful tool for both small and large-scale projects․ Key features include⁚
• Cross-Platform Compatibility⁚ CMake excels in its ability to generate build systems for a wide range of platforms‚ including Windows‚ macOS‚ Linux‚ and various embedded systems․
• Dependency Management⁚ CMake simplifies the process of managing external dependencies‚ allowing you to easily incorporate third-party libraries into your project․
• Build System Generation⁚ CMake generates native build systems‚ such as Makefiles or Visual Studio project files‚ tailored to the target platform․
• Testing Framework⁚ CMake provides a built-in testing framework that allows you to write and execute unit tests‚ ensuring the quality and reliability of your code․
• Package Management⁚ CMake supports package management‚ enabling you to easily distribute and install your software as a package‚ simplifying deployment and distribution․
• Extensibility⁚ CMake’s extensible nature allows you to customize its behavior and add new features through modules and custom commands․
Building‚ Testing‚ and Packaging with CMake
CMake streamlines the process of building‚ testing‚ and packaging C++ projects․ It automates these tasks‚ ensuring consistency and portability across different platforms․ Here’s how CMake simplifies these crucial aspects of software development⁚
• Building Applications⁚ CMake defines the compilation and linking process for your application‚ specifying compiler flags‚ linker settings‚ and dependencies․ It generates build scripts that can be executed on various platforms‚ ensuring a consistent build process․
• Testing Your Code⁚ CMake’s built-in testing framework enables you to write unit tests to verify the functionality and correctness of your code․ It facilitates the execution of these tests and provides detailed reports on test outcomes‚ helping you identify and fix bugs early in the development cycle․
• Packaging Your Software⁚ CMake aids in packaging your software for distribution․ It can generate installation packages‚ such as RPM or DEB packages‚ making it easy for users to install and use your application on various systems․ This simplifies deployment and ensures that your software is readily available to your users․
Building Applications
CMake simplifies the process of building C++ applications by automating the compilation and linking process․ You define the project’s structure‚ source files‚ dependencies‚ and build settings in a CMakeLists․txt
file․ CMake then interprets this file and generates platform-specific build scripts‚ such as Makefiles or Visual Studio project files․ These scripts handle the compilation of source code‚ linking of libraries‚ and creation of executables․
CMake’s ability to generate build scripts for various platforms ensures a consistent build process‚ regardless of the development environment․ This eliminates the need to manually configure build settings for each platform‚ saving time and effort․ CMake also allows you to define targets for different build configurations‚ such as debug or release builds‚ enabling you to tailor the build process to specific requirements․
Furthermore‚ CMake offers features like dependency management‚ allowing you to include external libraries and frameworks in your project․ It handles the necessary configuration and linking steps‚ ensuring that your application can utilize the required libraries seamlessly․
Testing Your Code
Modern CMake provides robust support for writing and running unit tests for your C++ code․ It integrates with popular testing frameworks like Google Test and Catch2‚ allowing you to seamlessly incorporate testing into your build process․ CMake enables you to define test targets that execute your tests as part of the build process‚ ensuring that your code is thoroughly tested before deployment․
CMake’s test facilities include features for generating test reports‚ allowing you to track the results of your tests and identify areas that require attention․ You can specify test dependencies‚ ensuring that tests are executed in the correct order and that any required prerequisites are met․ CMake also supports test filtering‚ enabling you to selectively run specific tests or test groups based on your needs․
By leveraging CMake’s testing capabilities‚ you can establish a comprehensive testing regimen for your C++ projects‚ enhancing the quality and reliability of your code․ This not only ensures that your code functions as intended but also helps you identify and fix bugs early in the development cycle‚ reducing the risk of introducing defects later in the process․
Packaging Your Software
Modern CMake simplifies the process of packaging your C++ software for distribution․ It provides tools for generating installation packages in various formats‚ such as ZIP archives‚ tarballs‚ and installers for different operating systems․ You can define the contents of your package‚ including executables‚ libraries‚ header files‚ and other supporting resources‚ and specify the target installation directory․
CMake supports creating self-contained packages that can be easily distributed and installed on different systems․ It allows you to include dependencies‚ ensuring that all necessary components are packaged together․ You can also configure the installation process‚ specifying the target system’s architecture‚ operating system‚ and other relevant settings․
Modern CMake’s packaging capabilities streamline the process of delivering your software to users‚ making it easier for them to install and use your applications․ By automating the packaging process‚ you can ensure consistency and reduce the risk of errors‚ ultimately improving the user experience and enhancing the professionalism of your software distribution․
Advanced CMake Techniques
Modern CMake empowers you with advanced techniques to handle complex scenarios in your C++ projects․ Mastering these techniques allows you to optimize build processes‚ manage dependencies effectively‚ and tailor your projects to specific environments․
One powerful technique is handling external dependencies․ CMake provides mechanisms to integrate third-party libraries into your project‚ ensuring they are correctly built and linked․ You can specify the location of these libraries‚ configure build options‚ and manage version conflicts․
Another advanced technique is conditional compilation․ CMake allows you to selectively compile code based on specific conditions‚ such as the target platform‚ compiler version‚ or build configuration․ This enables you to tailor your project to different environments and optimize performance for specific platforms․
By leveraging these advanced techniques‚ you can create highly adaptable and efficient C++ projects that seamlessly integrate with various libraries and environments․
External Dependencies
Modern CMake excels at managing external dependencies‚ which are essential for building complex C++ projects․ You can seamlessly integrate third-party libraries into your project‚ ensuring they are correctly built and linked․ CMake provides commands like find_package
to locate and configure dependencies‚ simplifying the process of incorporating libraries into your project․
For instance‚ when using a library like Boost‚ you can use find_package(Boost REQUIRED)
to locate the Boost library․ If found‚ CMake will set variables like Boost_INCLUDE_DIRS
and Boost_LIBRARIES
‚ which you can then use in your project․
This approach ensures that your project can easily incorporate external libraries‚ streamlining the build process and making your project more robust and adaptable․ Modern CMake simplifies dependency management‚ allowing you to focus on the core logic of your C++ application․
Conditional Compilation
Conditional compilation is a powerful technique for tailoring your C++ code to different environments or configurations․ Modern CMake provides a flexible way to control which parts of your code are compiled based on specific conditions․ This allows you to create more adaptable and modular projects‚ handling variations in platform‚ build settings‚ or feature availability․
CMake’s if
‚ elseif
‚ and else
commands enable you to define conditional blocks in your CMakeLists․txt files․ You can use these commands to check for specific features‚ such as the presence of a compiler flag‚ the target platform‚ or the existence of a particular library․
For example‚ you can use an if
statement to include a specific source file only when a certain compiler flag is defined․ This allows you to customize your project for different environments‚ ensuring that the correct code is compiled for each scenario․
Best Practices for CMake Projects
Crafting well-structured CMake projects is crucial for maintainability and scalability․ By adhering to best practices‚ you ensure that your projects are easy to understand‚ modify‚ and build across different platforms․
A well-defined project structure is the cornerstone of a successful CMake project․ Group related source files and headers logically into directories‚ mirroring the organization of your C++ code․ This promotes clarity and makes it easier to navigate your project․
Consistent code organization within your CMakeLists․txt files is equally important․ Employ meaningful variable names‚ utilize comments to explain complex logic‚ and break down large CMake files into smaller‚ more manageable modules․ This enhances readability and makes it easier for others to collaborate on your project․
Project Structure
A well-defined project structure is the cornerstone of a successful CMake project․ It promotes clarity‚ organization‚ and makes it easier to navigate your project․
A common and effective approach is to organize your project’s source code into logical directories․ For instance‚ you might have a “src” directory for source files‚ a “include” directory for header files‚ and a “tests” directory for unit tests․
Furthermore‚ consider creating separate directories for external dependencies‚ build artifacts‚ and documentation․ This separation ensures that your project’s structure remains clean and manageable‚ regardless of its size and complexity․
Code Organization
Proper code organization is paramount for maintaining a readable‚ scalable‚ and maintainable CMake project․ Employing good code organization practices can significantly reduce the cognitive load on developers‚ making it easier to understand and modify the project’s codebase․
One effective strategy is to group related source files and header files within dedicated directories․ This not only improves code clarity but also facilitates the creation of modular and reusable components․
Additionally‚ adopting consistent naming conventions for files‚ variables‚ and functions helps to ensure that your code remains easily understandable for both yourself and other developers working on the project․
Modern CMake in Action
To truly grasp the power and versatility of Modern CMake‚ it’s crucial to see it in action․ This section explores practical examples of how CMake can streamline your C++ development workflow․
From building simple console applications to complex libraries and frameworks‚ CMake provides the necessary tools to manage dependencies‚ configure build settings‚ and automate the entire build process․
The book “Modern CMake for C” delves into various real-world applications of CMake‚ showcasing how it can be used to create robust and maintainable projects․ These examples serve as valuable learning resources‚ demonstrating best practices and common patterns for utilizing CMake effectively in a variety of scenarios․
By exploring these examples‚ you’ll gain a deeper understanding of how CMake can be tailored to meet the specific needs of your C++ projects‚ ultimately enhancing your productivity and project quality․
Example Projects
To solidify your understanding of Modern CMake‚ “Modern CMake for C” provides a collection of comprehensive example projects․ These projects serve as practical demonstrations‚ showcasing how CMake can be applied in various scenarios․
From basic hello-world programs to more sophisticated libraries and applications‚ these examples cover a wide range of complexities․ By stepping through the CMake configurations and build processes‚ you gain valuable insights into best practices and common patterns for structuring your projects․
Each example project is carefully designed to illustrate specific CMake features‚ such as managing dependencies‚ setting up build configurations‚ and generating documentation․ You’ll discover how to use CMake to create clean‚ maintainable‚ and easily scalable projects․
These practical examples act as a springboard for your own projects‚ providing a solid foundation for applying Modern CMake techniques in your C++ development journey․
Real-World Applications
Modern CMake isn’t just a theoretical tool; it’s a powerful engine driving real-world software development․ From large-scale enterprise projects to open-source libraries‚ CMake is the backbone of countless C++ projects․
The “Modern CMake for C” guide highlights how CMake is used to build‚ test‚ and package software in various domains․ You’ll see examples of how CMake is employed in scientific computing‚ game development‚ embedded systems‚ and more․
By exploring these real-world applications‚ you gain a deeper understanding of how CMake is used to manage complex dependencies‚ configure build environments across different platforms‚ and streamline the development process․
You’ll also discover how CMake facilitates collaboration between developers‚ ensuring consistent builds and shared project configurations․ These examples demonstrate the versatility and practicality of Modern CMake in real-world software development scenarios․
Leave a Reply
You must be logged in to post a comment.